The Pipeline Pattern is a design pattern that is used to organize complex processes by breaking them down into a series of smaller, more manageable steps or stages. In software engineering, the Pipeline Pattern is commonly used for data processing, where data is passed through a series of stages, with each stage performing a specific task on the data before passing it on to the next stage.
The Pipeline Pattern is a powerful tool that can simplify complex processes, reduce the risk of errors, and increase the scalability of your code. In this article, we will discuss the Pipeline Pattern in detail, including its definition, benefits, and examples.
Definition of Pipeline Pattern
The Pipeline Pattern is a software design pattern that organizes complex processes into a series of smaller, more manageable stages. Each stage performs a specific task on the input data before passing it on to the next stage. The output of the last stage is the final result of the process.
The Pipeline Pattern can be implemented using a variety of techniques, including functions, classes, or even microservices. The key feature of the Pipeline Pattern is that it provides a clear and structured approach to complex data processing tasks.
How Does the Pipeline Pattern Work?
The Pipeline Pattern consists of several components that work together to achieve a specific goal. These components include:
- Input data: This is the data that needs to be processed. It could be in any format, such as text, images, or numbers.
- Stages: These are the individual components that perform specific functions on the input data. Each stage takes input data from the previous stage, processes it, and passes the output to the next stage. A stage can perform any kind of function, such as cleaning, filtering, or transforming data.
- Output data: This is the final result of the pipeline process. It could be in the same format as the input data or a completely different format, depending on the stages involved.
- Control Flow: This is the mechanism that coordinates the execution of the pipeline process. It determines the order in which the stages are executed and controls the flow of data between them.
Benefits of the Pipeline Pattern
There are several benefits to using the Pipeline Pattern:
- Modularity: By breaking down a complex task into smaller, more manageable steps, the Pipeline Pattern increases modularity. Each step can be developed and tested independently, making it easier to modify or replace individual steps without affecting the rest of the pipeline.
- Reusability: The Pipeline Pattern allows for the creation of reusable components that can be used in multiple pipelines. For example, a data cleansing step can be reused in multiple pipelines that require the same data cleansing logic.
- Ease of Maintenance: The Pipeline Pattern can make it easier to maintain code because it promotes code separation and modularity. This makes it easier to identify and fix issues when they arise.
- Parallelism: The Pipeline Pattern can be designed to take advantage of parallel processing, which can improve performance by processing multiple steps concurrently.
- Scalability: The pipeline can be designed to be scalable, allowing for the processing of large amounts of data or the addition of new processing stages as needed.
- Flexibility: The pipeline can be designed to be flexible, allowing for the modification of processing stages or the addition of new components without affecting the overall structure of the pipeline.
- Improved Performance: By breaking down complex processes into smaller stages, the Pipeline Pattern can improve performance by allowing each stage to operate independently and concurrently. This can lead to faster processing times and improved scalability.
- Better Error Handling: The Pipeline Pattern can improve error handling by isolating errors to individual stages. If an error occurs in one stage, the rest of the pipeline can continue to operate normally, reducing the risk of a catastrophic failure.
Examples of the Pipeline Pattern
Let’s take a look at some examples of the Pipeline Pattern in action.
1. Image Processing Pipeline
An image processing pipeline is a common example of the Pipeline Pattern in action. The pipeline may consist of several stages, including image resizing, color correction, and image enhancement. Each stage performs a specific task on the input image before passing it on to the next stage. The output of the last stage is the final processed image.
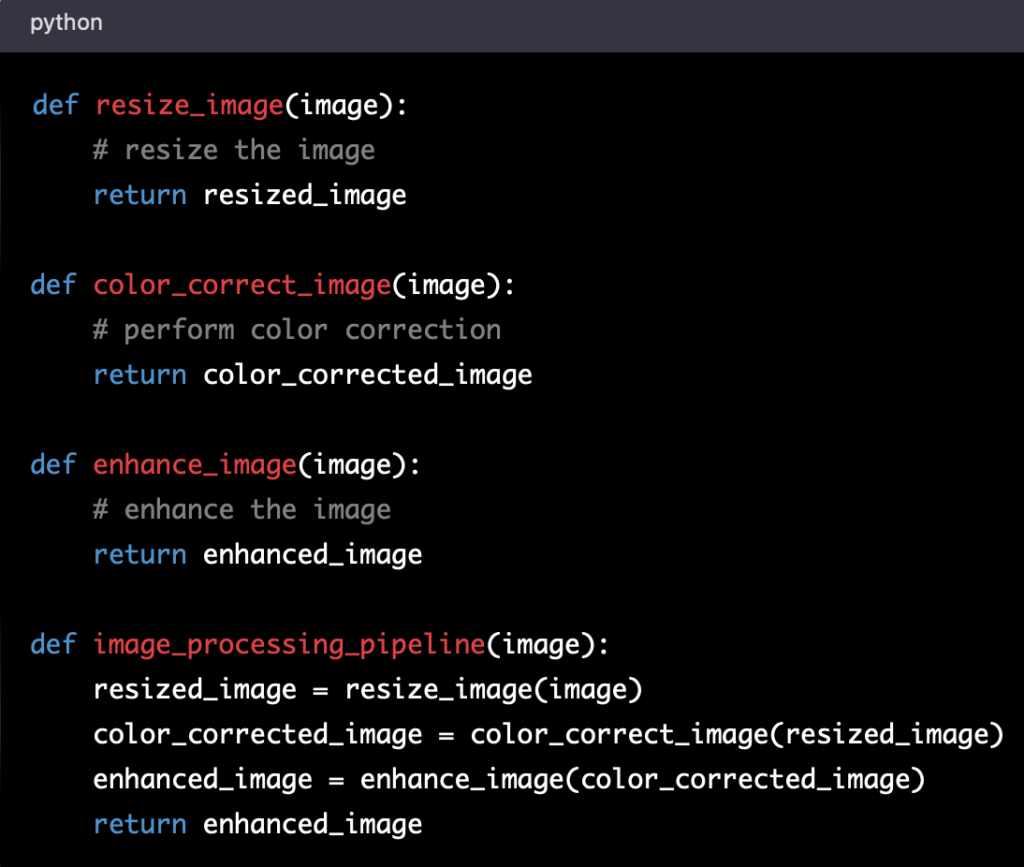
2. Text Processing Pipeline
Another example of the Pipeline Pattern is a text processing pipeline. The pipeline may consist of several stages, including text cleaning, tokenization, and feature extraction. Each stage performs a specific task on the input text before passing it on to the next stage. The output of the last stage is the final processed text.
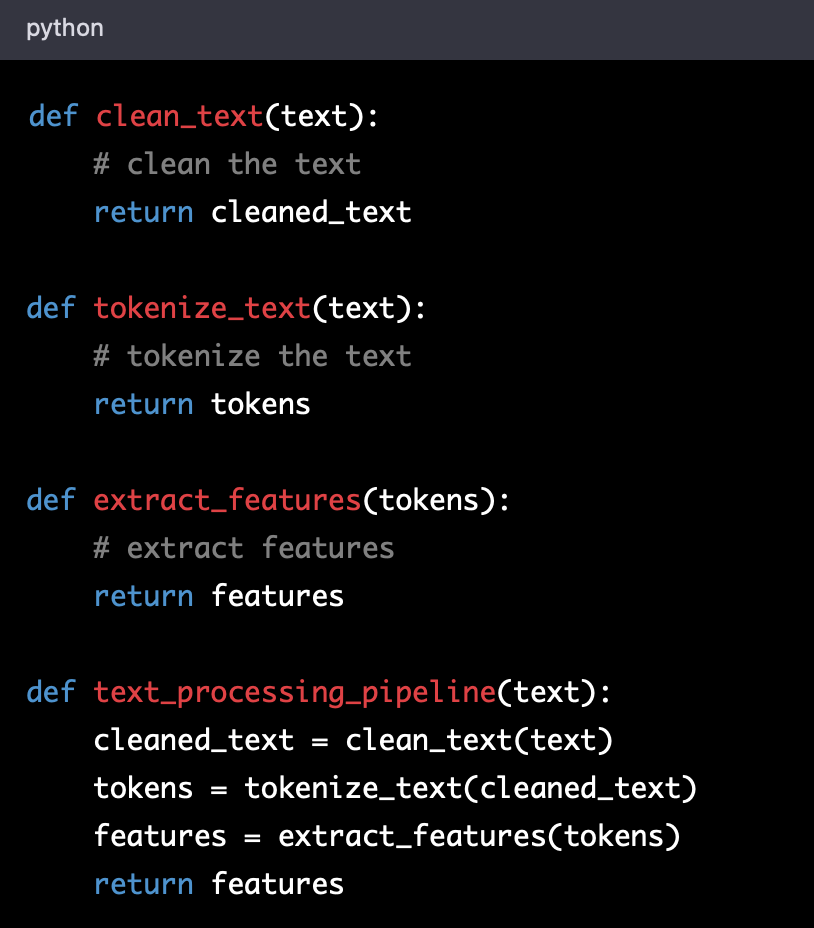